原文链接及内容
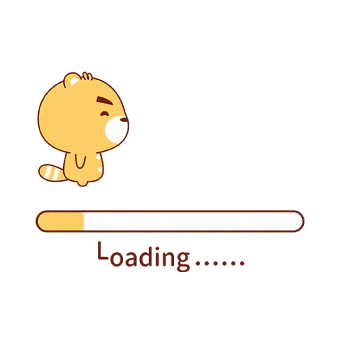
这里演示了ol/interaction/Draw
绘制交互的geometryFunction
选项的使用。从下拉菜单中选择一个几何形状类型开始绘制。要激活徒手绘图,请在绘制时按住Shift
键。正方形绘图是通过使用type: 'Circle'
类型和一个geometryFunction
来实现的,该函数会创建一个存在4条边的正多边形而不是一个圆。(方)框绘制也是使用type: 'Circle'
和一个geometryFunction
来实现的,该函数创建一个盒子形状的多边形而不是一个圆。同样地,绘制星星依旧使用了自定义几何函数,该函数使用绘制交互提供的中心和半径将圆形转换为星星形状。
main.js
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91
| import Draw, { createBox, createRegularPolygon, } from 'ol/interaction/Draw.js'; import Map from 'ol/Map.js'; import Polygon from 'ol/geom/Polygon.js'; import View from 'ol/View.js'; import {OSM, Vector as VectorSource} from 'ol/source.js'; import {Tile as TileLayer, Vector as VectorLayer} from 'ol/layer.js';
const raster = new TileLayer({ source: new OSM(), });
const source = new VectorSource({wrapX: false});
const vector = new VectorLayer({ source: source, });
const map = new Map({ layers: [raster, vector], target: 'map', view: new View({ center: [-11000000, 4600000], zoom: 4, }), });
const typeSelect = document.getElementById('type');
let draw; function addInteraction() { let value = typeSelect.value; if (value !== 'None') { let geometryFunction; if (value === 'Square') { value = 'Circle'; geometryFunction = createRegularPolygon(4); } else if (value === 'Box') { value = 'Circle'; geometryFunction = createBox(); } else if (value === 'Star') { value = 'Circle'; geometryFunction = function (coordinates, geometry) { const center = coordinates[0]; const last = coordinates[coordinates.length - 1]; const dx = center[0] - last[0]; const dy = center[1] - last[1]; const radius = Math.sqrt(dx * dx + dy * dy); const rotation = Math.atan2(dy, dx); const newCoordinates = []; const numPoints = 12; for (let i = 0; i < numPoints; ++i) { const angle = rotation + (i * 2 * Math.PI) / numPoints; const fraction = i % 2 === 0 ? 1 : 0.5; const offsetX = radius * fraction * Math.cos(angle); const offsetY = radius * fraction * Math.sin(angle); newCoordinates.push([center[0] + offsetX, center[1] + offsetY]); } newCoordinates.push(newCoordinates[0].slice()); if (!geometry) { geometry = new Polygon([newCoordinates]); } else { geometry.setCoordinates([newCoordinates]); } return geometry; }; } draw = new Draw({ source: source, type: value, geometryFunction: geometryFunction, }); map.addInteraction(draw); } }
typeSelect.onchange = function () { map.removeInteraction(draw); addInteraction(); };
document.getElementById('undo').addEventListener('click', function () { draw.removeLastPoint(); });
addInteraction();
|
界面布局文件index.html
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Draw Shapes</title> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css"> <link rel="stylesheet" href="node_modules/ol/ol.css"> <style> .map { width: 100%; height: 400px; } </style> </head> <body> <div id="map" class="map"></div> <div class="row"> <div class="col-auto"> <span class="input-group"> <label class="input-group-text" for="type">Shape type:</label> <select class="form-select" id="type"> <option value="Circle">Circle</option> <option value="Square">Square</option> <option value="Box">Box</option> <option value="Star">Star</option> <option value="None">None</option> </select> <input class="form-control" type="button" value="Undo" id="undo"> </span> </div> </div> <script src="https://cdn.jsdelivr.net/npm/elm-pep@1.0.6/dist/elm-pep.js"></script> <script type="module" src="main.js"></script> </body> </html>
|