原文链接及内容
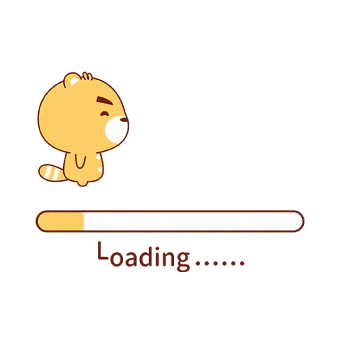
使用图标的宽度和高度属性的示例。用输入框中的值来调整图标实际的宽度和高度。
main.js
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87
| import Feature from 'ol/Feature.js'; import Map from 'ol/Map.js'; import Point from 'ol/geom/Point.js'; import TileJSON from 'ol/source/TileJSON.js'; import VectorSource from 'ol/source/Vector.js'; import View from 'ol/View.js'; import {Icon, Style} from 'ol/style.js'; import {Tile as TileLayer, Vector as VectorLayer} from 'ol/layer.js';
const widthInput = document.getElementById('width-input'); const heightInput = document.getElementById('height-input'); const clearWidthButton = document.getElementById('clear-width-button'); const clearHeightButton = document.getElementById('clear-height-button'); const scaleSpan = document.getElementById('scale');
const iconFeature = new Feature({ geometry: new Point([0, 0]), name: 'Null Island', population: 4000, rainfall: 500, }); const iconStyle = new Style({ image: new Icon({ src: 'data/icon.png', width: widthInput.value, height: heightInput.value, }), }); iconFeature.setStyle(iconStyle);
const image = iconStyle.getImage().getImage(); image.addEventListener('load', () => { scaleSpan.innerText = formatScale(iconStyle.getImage().getScale()); });
widthInput.addEventListener('input', (event) => { iconStyle.getImage().setWidth(event.target.value); iconFeature.changed(); scaleSpan.innerText = formatScale(iconStyle.getImage().getScale()); }); heightInput.addEventListener('input', (event) => { iconStyle.getImage().setHeight(event.target.value); iconFeature.changed(); scaleSpan.innerText = formatScale(iconStyle.getImage().getScale()); }); clearWidthButton.addEventListener('click', () => { widthInput.value = undefined; iconStyle.getImage().setWidth(undefined); scaleSpan.innerText = formatScale(iconStyle.getImage().getScale()); iconFeature.changed(); }); clearHeightButton.addEventListener('click', () => { heightInput.value = undefined; iconStyle.getImage().setHeight(undefined); scaleSpan.innerText = formatScale(iconStyle.getImage().getScale()); iconFeature.changed(); });
const vectorSource = new VectorSource({ features: [iconFeature], });
const vectorLayer = new VectorLayer({ source: vectorSource, });
const rasterLayer = new TileLayer({ source: new TileJSON({ url: 'https://a.tiles.mapbox.com/v3/aj.1x1-degrees.json?secure=1', crossOrigin: '', }), });
new Map({ layers: [rasterLayer, vectorLayer], target: document.getElementById('map'), view: new View({ center: [0, 0], zoom: 3, }), });
function formatScale(scale) { return Array.isArray(scale) ? '[' + scale?.map((v) => v.toFixed(2)).join(', ') + ']' : scale; }
|
界面布局文件index.html
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Icon Symbolizer width and height</title> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css"> <link rel="stylesheet" href="node_modules/ol/ol.css"> <style> .map { width: 100%; height: 400px; } </style> </head> <body> <div id="map" class="map"><div id="popup"></div></div>
<div class="controls"> <div style="margin: 1em 0;"> <label for="width">Width:</label> <input id="width-input" name="width" type="number" value="40"/> <button id="clear-width-button">clear</button> </div> <div style="margin: 1em 0;"> <label for="height">Height:</label> <input id="height-input" name="height" type="number" value="40"/> <button id="clear-height-button">clear</button> </div> <div style="margin: 1em 0;"> <label for="height">Scale approx.:</label> <span id="scale"></span> </div> </div> <script src="https://cdn.jsdelivr.net/npm/elm-pep@1.0.6/dist/elm-pep.js"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js"></script> <script type="module" src="main.js"></script> </body> </html>
|