原文链接及内容
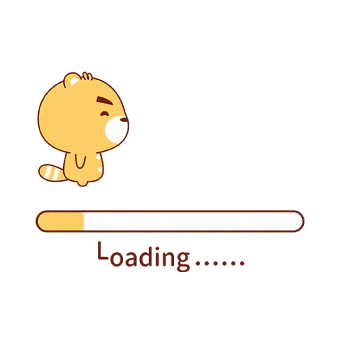
源代码
main.js
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| import Map from 'ol/Map.js'; import OSM from 'ol/source/OSM.js'; import TileLayer from 'ol/layer/Tile.js'; import View from 'ol/View.js';
const map = new Map({ layers: [ new TileLayer({ source: new OSM(), }), ], target: 'map', view: new View({ center: [0, 0], zoom: 2, }), });
document.getElementById('zoom-out').onclick = function () { const view = map.getView(); const zoom = view.getZoom(); view.setZoom(zoom - 1); };
document.getElementById('zoom-in').onclick = function () { const view = map.getView(); const zoom = view.getZoom(); view.setZoom(zoom + 1); };
|
界面布局文件index.html
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Accessible Map</title> <link rel="stylesheet" href="node_modules/ol/ol.css"> <style> .map { width: 100%; height: 400px; } a.skiplink { position: absolute; clip: rect(1px, 1px, 1px, 1px); padding: 0; border: 0; height: 1px; width: 1px; overflow: hidden; } a.skiplink:focus { clip: auto; height: auto; width: auto; background-color: #fff; padding: 0.3em; } #map:focus { outline: #4A74A8 solid 0.15em; } </style> </head> <body> <a class="skiplink" href="#map">Go to map</a> <div id="map" class="map" tabindex="0"></div> <button id="zoom-out">Zoom out</button> <button id="zoom-in">Zoom in</button> <script src="https://cdn.jsdelivr.net/npm/elm-pep@1.0.6/dist/elm-pep.js"></script> <script type="module" src="main.js"></script> </body> </html>
|
实践
将map
所在的div
元素的tabindex
属性设置为0
,使得地图可被聚焦。要使地图元素成为焦点,你可以单击键盘上的Tab
键导航到它,也可以使用跳转链接,详见index.html
的<a>
超链接标签,设置它的href
属性为map
所在div
的id
属性)具体操作为:
- 方式1:点击超链接即可跳转至地图;
- 方式2:通过按
Tab
键将焦点聚焦至超链接元素,按下Enter
键则焦点会自动聚焦至map
所在div
元素。
- 方式3:直接点击地图(哈哈这个好像是废话)
具体地,请看如下演示(屏幕下方有按键提示):
这里地图并没有全屏,方便观察焦点所在,其实我们第一次点击地图时,焦点就落到地图元素上了,其实这个tabindex
属性只是针对视障用户的一些贴心的设置。
细心的话会发现地址栏会有一个锚点,这个便是在超链接设置的href
的属性:
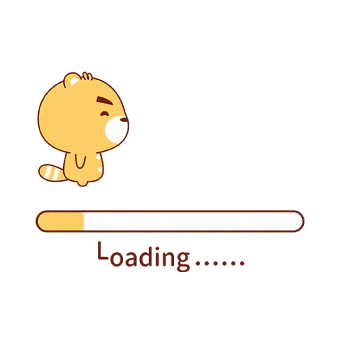
另外,当聚焦至地图元素时,+
和-
键可用于放大和缩小,箭头
键(即键盘上的方向键)可用于平移。
注:键盘上的+
需要同时按住Shift
键,-
则不需要。
点击地图下方的Zoom in(放大)
和Zoom out(缩小)
按钮可以放大和缩小地图。你也可以使用Tab
键导航至按钮,然后按Enter
键触发缩放操作。如下所示:我们将超链接移除,则按一下Tab
键即可跳转至map
元素。
演示的代码结合源码修改如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93
| <template> <div class="wrapper"> <div id="map" ref="map" tabindex="0"></div> <div class="handle"> <el-button class="zoom-out" @click="zoomOut">缩小</el-button> <el-button class="zoom-in" @click="zoomIn">放大</el-button> </div> </div> </template>
<script> import { Map, View } from 'ol'; import { Tile as TileLayer } from 'ol/layer'; import { fromLonLat } from 'ol/proj'; import { OSM } from 'ol/source';
export default { name: "MapView", data() { this.map = null; return {
}; }, mounted() { this._createBasemap(); }, methods: {
_createBasemap() { const layer = new TileLayer({ source: new OSM(), })
const mapView = new View({ center: fromLonLat([113.918, 35.308]), zoom: 8, });
this.map = new Map({ target: this.$refs.map, layers: [layer], view: mapView, }); }, zoomOut() { const view = this.map.getView(); const zoom = view.getZoom(); view.setZoom(zoom - 1); }, zoomIn() { const view = this.map.getView(); const zoom = view.getZoom(); view.setZoom(zoom + 1); }, }, } </script>
<style lang="scss" scoped> .wrapper { height: 100%; margin: 0; padding: 0; text-align: center;
a.skiplink { display: inline-block; background-color: white; padding: 10px; margin-top: 10px; }
#map { width: 60%; height: 60%; margin: 20px auto; padding: 0; border: 3px solid transparent;
&:focus{ border: 3px solid red; } }
.handle { margin: 30px auto; } } </style>
|
额外地
关于accessible
为何翻译为无障碍,最开始我的确用有道查询过,如可翻译为:可接近的,可访问的,但感觉并不符合示例所要表达的意思,后来想到之前在it之家读到的一片科普文章,感觉翻译为无障碍更恰如其分,关于无障碍方面的东西,大家也可以了解一下,这个也涉及到前端开发的一些知识,先看一张截图:
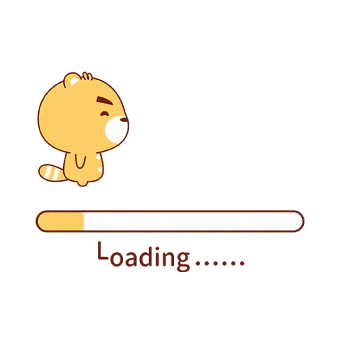
更多关于无障碍
方面的内容详见【IT之家学院】改善视障用户的网页体验就这么简单